Python is one of the most commonly use scripting tools. As a cyber security professional it is always good to have a few scripting language up your sleeve. It is always important to be able to understand simple logs and use a scripting tool to get high level understanding of the threats that might be happening in your environment.
In this tutorial we will cover how you can use python to open the files that we have use grep to filter out the source IP address of potential brute force attacks.
To understand the basic syntax. You can go to website like W3Schools to learn about the basics of Python.
Below is a list of mode for file operations.
Mode | Descriptions |
‘r’ | Opens a file for reading. (default) |
‘w’ | Opens a file for writing. Creates a new file if it does not exists and overwrite the file if it exists. |
‘a’ | Open a file and append any additional input. If file does not exists it creates a new file. It does not overwrite the file. |
‘x’ | Opens a file for exclusive creation. If the file already, the operation fails. |
‘t’ | Opens in text mode. (default) |
‘b’ | Opens a file in binary mode. |
‘+’ | Opens a file for updating (reading and writing) e.g ‘r+’ |
Reading from file (draco_ip.txt) and printing its content to console. By default the open module allows you to read the file.
with open(‘draco_ip.txt’) as draco_ip_file:
ip_address = draco_ip_file.read()
print (ip_address)
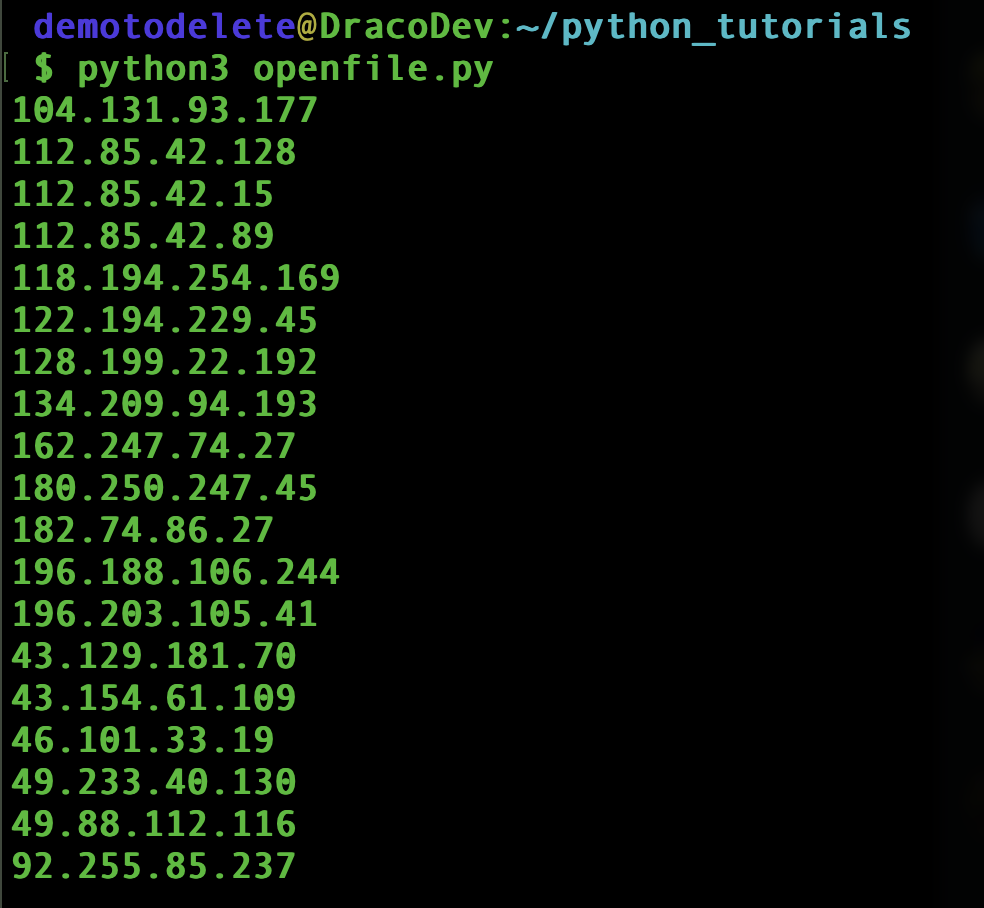
Next let’s look at reading from a file (draco_ip.txt) and writing to a new file (new_draco_ip.txt) with a comment added to the top of the file.
#!/usr/bin/python3
# open and read from the file draco_ip.txt and print output to console
with open(‘draco_ip.txt’) as draco_ip_file:
ip_address = draco_ip_file.read()
print (ip_address)
# assign a string to the variable comments to write to a new file
comments = (“IP Address of brute force source”)
”’
Multi-line DESCRIPTIONS
open file new_draco_ip.txt and overwrite the content with comments and ip_address.
if file not found create a new file with the name new_draco_ip.txt
use the .write() method to write to file
”’
with open(‘new_draco_ip.txt’,’w’) as draco_ip_write_to:
draco_ip_write_to.write(comments + ‘\n’ + ip_address)
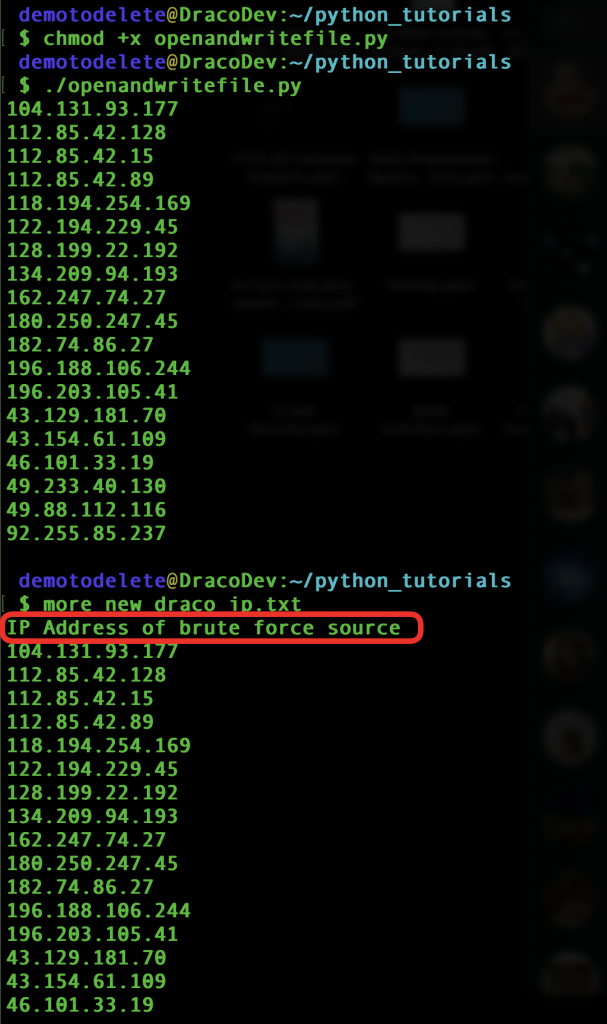
To append a comment to the end of the file.
append_to_end_comments = (“Append to the end”)
with open(‘new_draco_ip.txt’,’a’) as draco_ip_append_to:
draco_ip_append_to.write(append_to_end_comments)
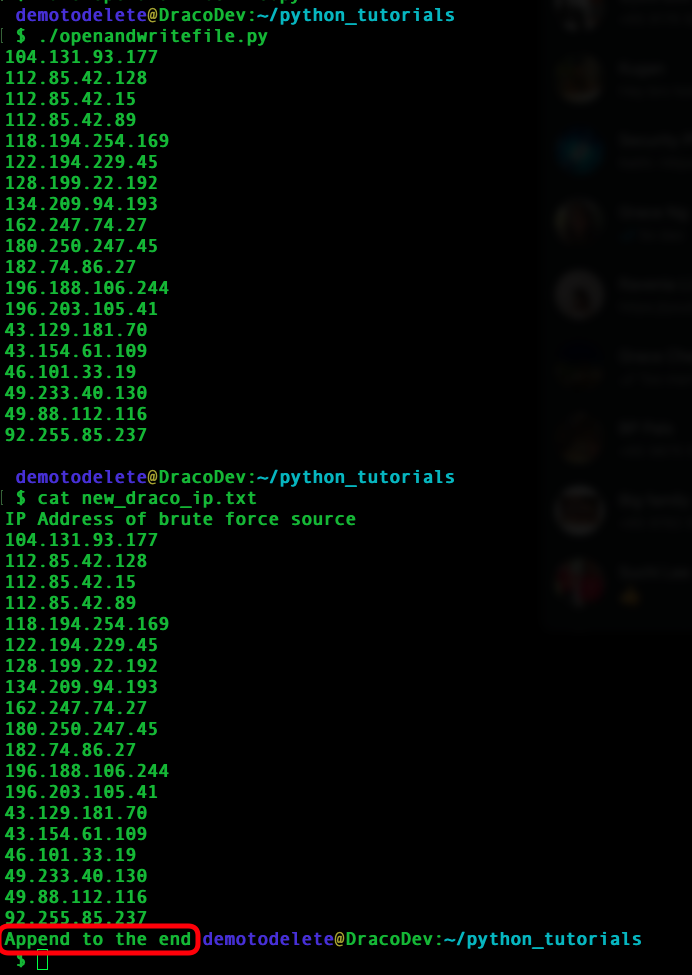
Using try and except to catch error when opening file.
# using try to catch error and print a error msg
try:
# open and read from the file draco_ip.txt and print output to console
with open(‘draco_ip.txt’) as draco_ip_file:
ip_address = draco_ip_file.read()
except FileNotFoundError:
error_msg = “Sorry file not found”
print(error_msg)
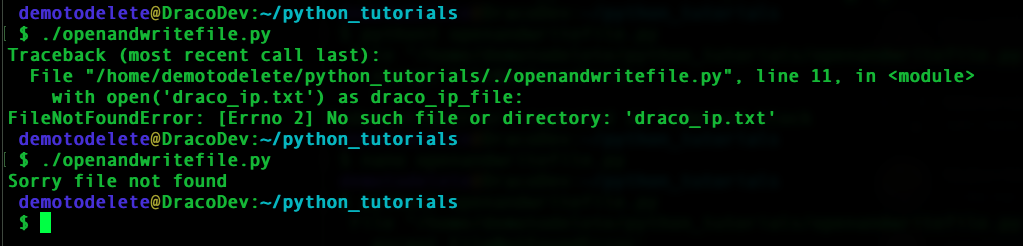
Below are a list of methods that can be use with file operation in text mode for the your reference.
Method | Descriptions |
close() | Close an open file. |
detach() | Separates the underlying binary buffer from the TextIOBase and retun it |
fileno() | Returns an integer number (file descriptor) of the file. |
flush() | Flushes the write buffer of the file stream. |
isatty() | Returns True if the file stream is interactive. |
read(n) | Read at most n (number) of characters from the file. If no value specific read till end of file. |
readable() | Return True if the file stream can be read from. |
readline(n=-1) | Read at most n (number of bytes) from line. If no value specific read the entire line. |
readlines(n=-1) | Read and return a list of lines from the file. Read in at most n bytes/characters if specified. |
seekable() | Returns True if the file stream supports random access. |
tell() | Returns an integer that represents the current position of the file’s object |
truncate(size=None) | Resizes the file stream to size bytes. If size is not specified, resizes to current location. |
writeable() | Return True if the file stream can be written to. |
write(str) | Writes the string str to the file and returns the number of characters written. |
writelines(lines) | Write a list of lines to the file. |
Hope this short tutorial is useful for your lab testing.